byMarc.JS.dropDownCheckBoxList |
A pure javascript drop-down checkbox list control for web developers. |
|
Sample1 - simple demo with 6 items
|
|
Table of Contents: |
|
- Features |
|
- Usage Syntax |
|
- Minimum and Maximum Required Selections |
|
- Dynamic Selection Display |
|
- All and No Selections Text |
|
- Fixed Header Text |
|
- Auto Sort |
|
- Vertical Scrolling |
|
- Click Outside Auto Cancel |
|
- Cancel Selection Restoration |
|
- Select and Deselect All |
|
- Disabled Select List Items |
|
- Parent Container |
|
- Options |
|
- State Properties |
|
- CSS Styling |
|
- CSS Styles |
|
- Button Classes |
|
- NuGet Packaging |
|
- HTML Integration |
|
|
|
Features: |
|
- allows the user to expand and collapse the checkbox list window, |
|
- uses a check box list to allow the user to select and deselect multiple options, |
|
- selections can be cancelled--restoring the previous selections, |
|
- clicking outside the drop-down cancels and closed the drop-down automatically, |
|
- applications can require the user to select a minimum number of items and not more than a maximum number of items, |
|
- items can be sorted by the control, if desired, |
|
- key elements have class names assigned to control various visual aspects of the control, |
|
- the control can display fixed text or a comma delimited list of selected values, |
|
- supports multiple controls on the same page, |
|
- automatic vertical scrolling, |
|
- auto cancel on click outside drop-down, |
|
|
|
|
Usage Syntax: |
|
To create an instance of the control, the application must call the javascript function createDropDownCheckBoxList. The function accepts a single anonymous parameter. See the section Options, for specific details on each available option. The function will return an HTML element with an instance of the control. The application can then append the control as a child to any element in the DOM. Also, for convenience, the createDropDownCheckBoxList function can append the control to any element by using the parentElement option. |
|
|
function createDropDownCheckBoxList(data) |
|
|
|
Sample2 - minimalist demo with no options*
|
|
Minimum and Maximum Required Selections: |
|
The control allows the application to specify a minimum and a maximum number of selections that the user is required to make. This demo forces the user to select between 1 and 3 colors. If the required number of selections hasn't been made, the Ok button is disabled. |
|
|
|
Sample3 - demo requiring between 1 and 3 selections*
|
|
Dynamic Selection Display: |
|
By default, the control typically displays the selection titles as they are being made. The display will automatically display an ellipsis when the width of the selections exceeds the available space. |
|
All and No Selections Text: |
|
In dynamic selection display mode, the control can optionally display "special" values when all or none of the select list items are selected (including disabled items). A checked disabled item will prevent the noSelectionsText value from being displayed and in contrast, an unchecked disabled item will prevent the allSelectionsText from being displayed. |
|
|
|
Sample4 - demo with special selection count text*
|
|
Fixed Header Text: |
|
The control can optionally disable the dynamic selection display and simply display a static header. |
|
|
|
|
Sample5 - demo of a fixed header text*
|
|
Auto Sort: |
|
The control can automatically sort the select list items. By default, the control does not sort the items. |
|
|
|
Sample6 - auto sorting demo*
|
|
Vertical Scrolling: |
|
By default, the drop-down will automatically increase in size with the number of select list items. By apply a height to the CSS style, the drop-down height can be fixed. When the number of select list items exceeds the available drop-down height, a scroll bar will appear automatically. For more information see the scrollDiv style in the CSS Styling section. |
|
|
|
Sample7 - vertical scrolling enabled demo*
|
|
Click Outside Auto Cancel: |
|
The control is designed to automatically cancel the drop-down, when the user clicks anywhere outside of the control. This feature provides the control a somewhat modal functionality. It also assists in the UI by automatically closing the windows when the user expands a different control. |
|
Cancel Selection Restoration: |
|
The drop-down window includes a cancel button. When pressed, the previous selections are automatically restored. |
|
Select and Deselect All: |
|
The drop-down window automatically includes as the first select item a special checkbox that can select or deselect all of the items. |
|
Disabled Select List Items: |
|
The control is capable of rendering select list items disabled by setting the "Disabled" property on specific select list items. The application can specify whether a disabled select list item is checked or not. Disabled select list items perform exactly the same way as non-disabled items except that the user will not be able to check or uncheck disabled item. The "Select All" feature cannot be used to check or uncheck disabled items. When a select list item is disabled, the control appends the byMarc-ddcbl-checkbox-disabled to the checkbox element and the byMarc-ddcbl-itemlabel-disabled to the label element. |
|
|
|
Sample8 - disabled items demo*
|
|
|
|
|
Sample9 - disabled selections with 2 or 3 required selections demo*
|
|
Parent Container: |
|
When the control generates the required DOM, it can optionally append the control to a parent container element. This can be accomplished using the parentElement option. If an element is provided in the parentElement property, the control will be appended to it; otherwise, if the parentElement is not null the control will perform a getElementById to retrieve an element from the DOM using the value of the parentElement option. If found, the control will be appended to it. |
|
|
|
Options: |
|
The control accepts an options anonymous parameter. These options control various features of the control. The following options are supported: |
|
|
|
Property |
Data Type |
Description |
name
|
string |
The name that is assigned to the select list items when rendered. |
onOkClick |
string |
Javascript to execute when the |
selectListItems |
{}[] |
The select list items to be displayed. It is an array of anonymous class objects with the following properties:
Property |
Data Type |
Description |
Text |
string |
This is the text to display. |
Value |
string |
This is the value of the item. |
Selected |
boolean |
True, to initially set the item as checked; false to initially set as unchecked. |
Disabled |
boolean |
True, to disable the select list item; false, otherwise. |
|
minSelections |
number |
Specifies the minimum number of selections required before the Ok button is enabled. |
maxSelections |
number |
Specifies the maximum number of selections allowed before the Ok button is disabled. |
noSelectionsText |
string |
The text to display, when there are no items selected. Ignored, if fixedHeaderText is specified. |
allSelectionsText |
string |
The text to display, when all item are selected. Ignored, if fixedHeaderText is specified. |
parentElement |
html element or string |
If null, the control will not be added as a child to any elements. If a string is specified, a call to getElementByID will be used to retrieve an element from the DOM and this property will be updated to a reference of the element; otherwise, a reference to an html element is required. |
autoSort |
boolean |
True, for the control to sort the select list items; false, otherwise. False, by default. |
fixedHeaderText |
string |
If specified, the display will always show this value regardless of the selected items. |
|
|
State Properties: |
|
The control uses an anonymous object to maintain state. The table below identifies the variables used: |
|
|
|
Property |
Data Type |
Description |
selectedCount |
number |
Indicates the number of items selected. |
displayElement |
html element |
The element used to display the value. |
selectAllElement |
html element |
The (Select All) select list item. |
buttonElement |
html element |
The element used for the button. |
isCollapsed |
boolean |
Indicates the state of the drop-down. |
restoreValue |
string |
Stores the value to be restored, if the drop-down is cancelled. |
dropDownElement |
html element |
The drop-down element that is hidden and shown. |
element |
html element |
The top-most element that contains the entire control. |
okButtonElement |
html element |
The Ok button element. |
cancelButtonElement |
html element |
The Cancel button element. |
|
|
|
CSS Styling: |
|
The control uses CSS to style the control and to also support application specific configuration. By making use of CSS selectors and the parent element ID, CSS styling can be selectively applied to one or more controls. See the Vertical Scroll Bar section for a sample of this feature. |
|
|
|
#sample7 .byMarc-ddcbl-scrollDiv {
height: 50px;
} |
|
|
CSS Styles: |
|
The following table below identifies the class names used by the control. |
|
|
|
Style |
Description |
byMarc-ddcbl-table |
The top-most table. |
byMarc-ddcbl-commandsTableRow |
The <tr> used to contain the Ok and the Cancel buttons. |
byMarc-ddcbl-displayDiv |
The <div> used to display the value. It provide the container functionality so that the inner <span> element can render an ellipsis when needed. |
byMarc-ddcbl-item |
The <td> elements that contain the checkboxes. |
byMarc-ddcbl-itemRow |
The <tr> element that contains a select list item. |
byMarc-ddcbl-checkbox-disabled |
The class that is added to the checkbox element when the select list item is disabled. |
byMarc-ddcbl-checkbox |
Each checkbox <input> element. |
byMarc-ddcbl-itemlabel |
The <td> elements that contain the labels for each select list item. |
byMarc-ddcbl-itemlabel-disabled |
The class that is added to the <td> element for a select list item label when the select list item is disabled. |
byMarc-ddcbl-commandsTableCell |
The <td> element that contains the Ok and Cancel buttons. |
byMarc-ddcbl-command |
The <input> elements for the Ok and Cancel buttons. |
byMarc-ddcbl-command-disabled |
The class that is added to the <input> Ok button when the Ok is disabled. |
byMarc-ddcbl-dropdown |
The drop-down <table> element that is hidden and shown on demand. |
byMarc-ddcbl-inputbox |
The <tr> element that holds the display and the drop-down button. |
byMarc-ddcbl-input |
The <span> element that displays the ellipsis when needed. |
byMarc-ddcbl-scrollDiv |
This style is applied to the <div> control that contains all of the select list items. This is also the control that will provide the vertical scrolling functionality. By default, no height is applied. See the Vertical Scrolling section for more details. By appling a height or a max-height style, the scrolling functionality can be activated. |
|
|
|
Button Classes: |
|
In addition to the CSS styles above, the drop-down button is assigned a class name depending on the current state of the control. There are 2 internal properties (see the State Properties section for more details) that determine the corresponding state: isCollapsed, selectedCount. The table below indicates which class names are assigned for each state. The button element will be assigned five class names--one from each group below: |
|
|
|
Class Name |
Collapsed |
Expanded |
All Items Selected |
No Items Selected |
Some Items Selected |
byMarc-ddcbl-button |
Yes |
Yes |
Yes |
Yes |
Yes |
|
|
|
|
|
|
byMarc-ddcbl-button-collapsed |
Yes |
|
Yes |
Yes |
Yes |
byMarc-ddcbl-button-expanded |
|
Yes |
Yes |
Yes |
Yes |
|
|
|
|
|
|
byMarc-ddcbl-button-collapsed-all |
Yes |
|
Yes |
|
|
byMarc-ddcbl-button-collapsed-some |
Yes |
|
|
|
Yes |
byMarc-ddcbl-button-collapsed-none |
Yes |
|
|
Yes |
|
byMarc-ddcbl-button-expanded-all |
|
Yes |
Yes |
|
|
byMarc-ddcbl-button-expanded-some |
|
Yes |
|
|
Yes |
byMarc-ddcbl-button-expanded-none |
|
Yes |
|
Yes |
|
|
|
|
|
|
|
byMarc-ddcbl-button-all |
Yes |
Yes |
Yes |
|
|
byMarc-ddcbl-button-some |
Yes |
Yes |
|
|
Yes |
byMarc-ddcbl-button-none |
Yes |
Yes |
|
Yes |
|
|
|
|
|
|
|
byMarc-ddcbl-button-collapsed-notAll |
Yes |
|
|
Yes |
Yes |
byMarc-ddcbl-button-collapsed-notSome |
Yes |
|
Yes |
Yes |
|
byMarc-ddcbl-button-collapsed-notNone |
Yes |
|
Yes |
|
Yes |
byMarc-ddcbl-button-expanded-notAll |
|
Yes |
|
Yes |
Yes |
byMarc-ddcbl-button-expanded-notSome |
|
Yes |
Yes |
Yes |
|
byMarc-ddcbl-button-expanded-notNone |
|
Yes |
Yes |
|
Yes |
|
|
|
|
|
|
byMarc-ddcbl-button-notAll |
Yes |
Yes |
|
Yes |
Yes |
byMarc-ddcbl-button-notSome |
Yes |
Yes |
Yes |
Yes |
|
byMarc-ddcbl-button-notNone |
Yes |
Yes |
Yes |
|
Yes |
|
|
NuGet Packaging: |
|
The latest version of the NuGet package can be found here. |
|
HTML Integration: |
|
Install the latest version of the NuGet package. Three byMarc/dropDownCheckBoxList folders should appear in the Content, Images and Scripts folders. They should contain the .css, .png and .js files. |
|
|
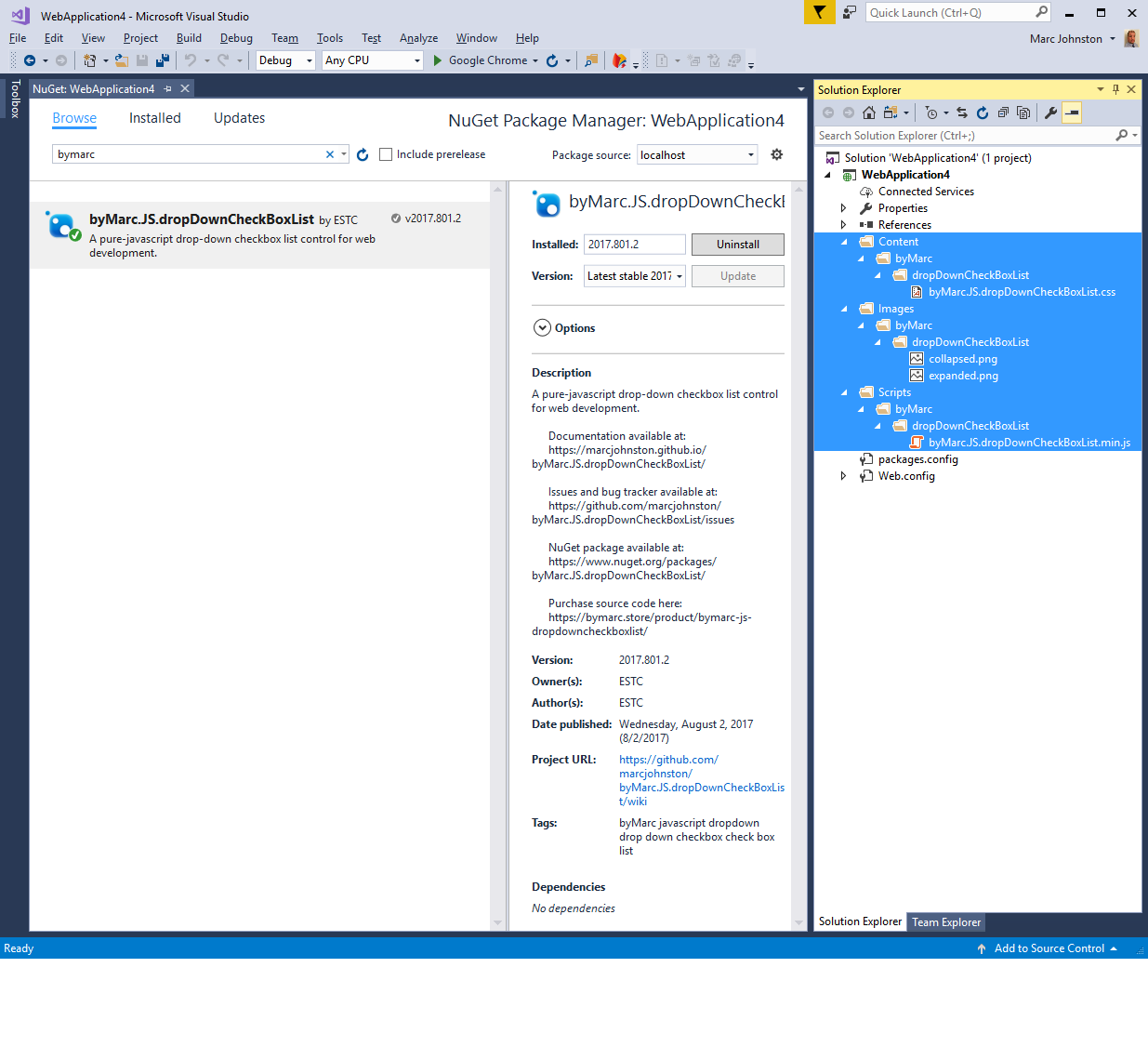 |
|
To use the control on a page, links to the .js and .css styles are needed.
|
|
<link href="Content/byMarc/dropDownCheckBoxList/byMarc.JS.dropDownCheckBoxList.css" rel="stylesheet" />
<script src="Scripts/byMarc/dropDownCheckBoxList/byMarc.JS.dropDownCheckBoxList.min.js"></script>
|
|
|
|
Create a <div> element with an ID. This element will be used as the parent container element. |
|
<div id="dropDownCheckBoxList"></div> |
|
|
|
Instances of the control can be created by calling the createDropDownCheckBoxList javascript method. To seat the control, supply the parentElement option. |
|
<script>createDropDownCheckBoxList({ parentElement: dropDownCheckBoxList })</script> |
|
|
|
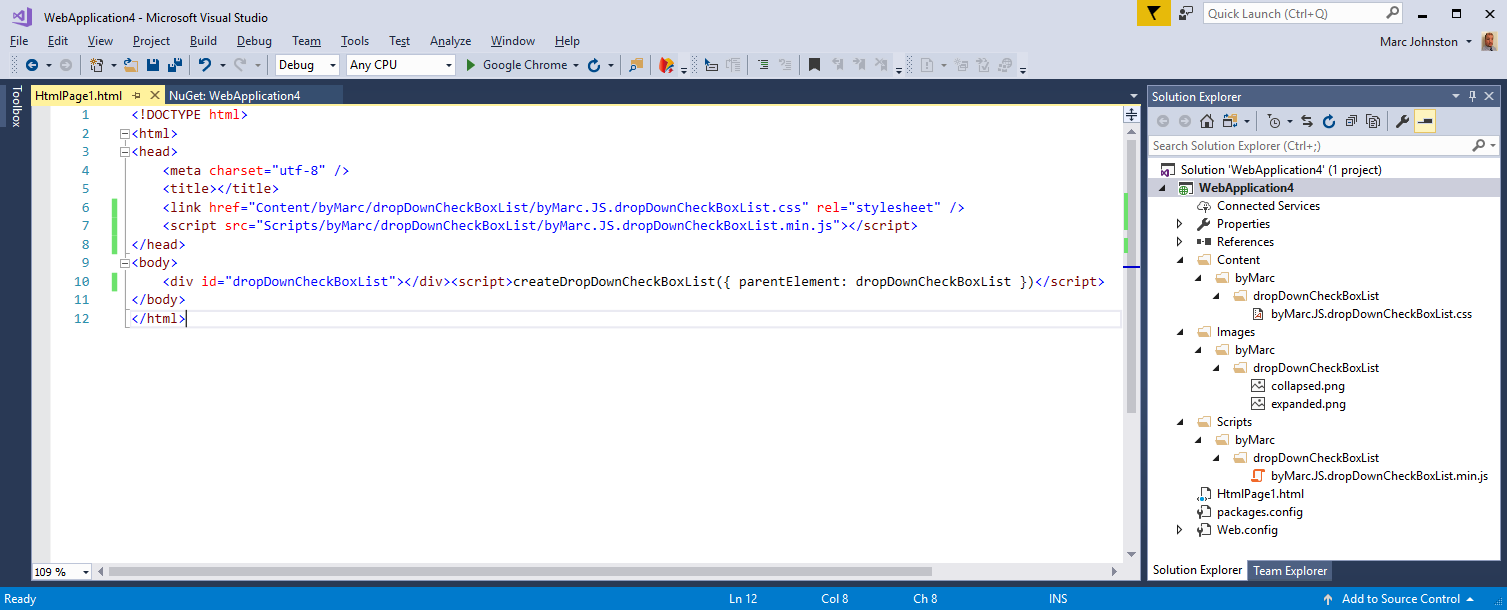 |
|
|
The drop-down should by working: |
|
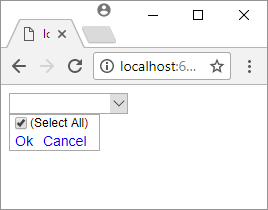 |
|
|
|
byMarc is a registered trademark by Enterprise Solutions & Technology Consulting. All rights reserved. This documentation created using Adobe Dreamweaver. |